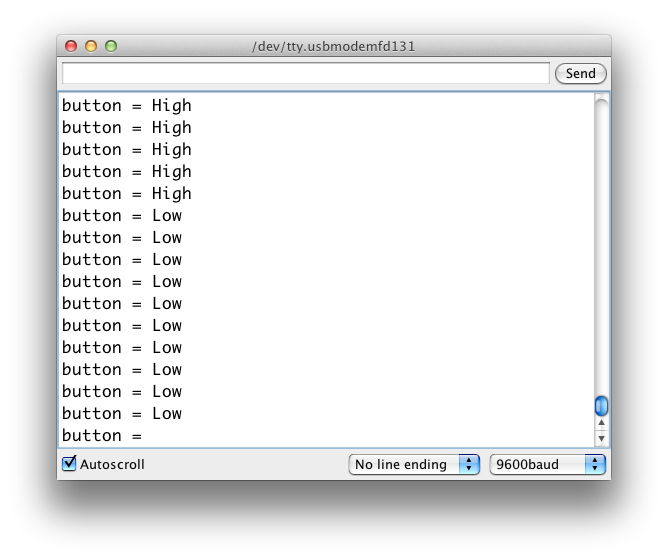
// Arduino ----------------------------------------------
// simpleTweet_00_a
/*
simpleTweet_00 arduino sketch (for use with
simpleTweet_00 processing sketch) by @msg_box june2011
This script is intended for use with a magnetic reed switch,
but any on/off switch plugged into pin #10 will readily work.
The Arduino is connected to a circuit with a sensor that
triggers the code: Serial.write(n); where n = 1 or 2.
The Processing sketch listens for that message and then
uses the twitter4j library to connect to Twitter
via OAuth and post a tweet.
To learn more about arduino, processing, twitter4j,
OAuth, and registering your app with Twitter...
visit <http://www.instructables.com/id/Simple-Tweet-Arduino-Processing-Twitter/>
visit <http://www.twitter.com/msg_box>
This code was made possible and improved upon with
help from people across the internet. Thank You.
Special shoutouts to the helpful lurkers at twitter4j,
arduino, processing, and bloggers everywhere, and
to the adafruit & ladydada crowdsource.
And above all, to my lovely wife, without
whom, none of this would have been possible.
Don't be a dick.
*/
const int magReed_pin = 2; // pin number
const int ledPin = 13;
int magReed_val = 0;
int currentDoorState = 1; // begin w/ circuit open
int previousDoorState = 1;
void setup(){
Serial.begin(9600);
pinMode(magReed_pin, INPUT);
pinMode(ledPin, OUTPUT);
}
void loop(){
watchTheDoor();
}
void watchTheDoor(){
magReed_val = digitalRead(magReed_pin);
if (magReed_val == LOW){ // open
currentDoorState = 1;
digitalWrite(ledPin, LOW);
}
if (magReed_val == HIGH){ // closed
currentDoorState = 2;
digitalWrite(ledPin, HIGH);
}
compareStates(currentDoorState);
}
void compareStates(int i){
if (previousDoorState != i){
previousDoorState = i;
Serial.write(i);
delay(1000); //
}
}
// Processing -------------------------------
import processing.serial.*;
import twitter4j.conf.*;
import twitter4j.internal.async.*;
import twitter4j.internal.org.json.*;
import twitter4j.internal.logging.*;
import twitter4j.http.*;
import twitter4j.api.*;
import twitter4j.util.*;
import twitter4j.internal.http.*;
import twitter4j.*;
static String OAuthConsumerKey =
static String OAuthConsumerSecret =
static String AccessToken =
static String AccessTokenSecret =
Serial arduino;
Twitter twitter = new TwitterFactory().getInstance();
void setup() {
size(125, 125);
frameRate(10);
background(0);
println(Serial.list());
String arduinoPort = Serial.list()[4]; // Your Serial Port !!!
arduino = new Serial(this, arduinoPort, 9600);
loginTwitter();
}
void loginTwitter() {
twitter.setOAuthConsumer(OAuthConsumerKey, OAuthConsumerSecret);
AccessToken accessToken = loadAccessToken();
twitter.setOAuthAccessToken(accessToken);
}
private static AccessToken loadAccessToken() {
return new AccessToken(AccessToken, AccessTokenSecret);
}
void draw() {
background(0);
text("iLMS Tweet", 15, 45);
text("@bemoredev", 30, 70);
listenToArduino();
}
void listenToArduino() {
String msgOut = "";
int arduinoMsg = 0;
if (arduino.available() >= 1) {
arduinoMsg = arduino.read();
if (arduinoMsg == 1) {
msgOut = "LED1 On at "+hour()+":"+minute()+":"+second();
}
if (arduinoMsg == 2) {
msgOut = "LED1 Off at "+hour()+":"+minute()+":"+second();
}
compareMsg(msgOut); // this step is optional
// postMsg(msgOut);
}
}
void postMsg(String s) {
try {
Status status = twitter.updateStatus(s);
println("new tweet --:{ " + status.getText() + " }:--");
}
catch(TwitterException e) {
println("Status Error: " + e + "; statusCode: " + e.getStatusCode());
}
}
void compareMsg(String s) {
// compare new msg against latest tweet to avoid reTweets
java.util.List statuses = null;
String prevMsg = "";
String newMsg = s;
try {
statuses = twitter.getUserTimeline();
}
catch(TwitterException e) {
println("Timeline Error: " + e + "; statusCode: " + e.getStatusCode());
}
Status status = (Status)statuses.get(0);
prevMsg = status.getText();
String[] p = splitTokens(prevMsg);
String[] n = splitTokens(newMsg);
//println("("+p[0]+") -> "+n[0]); // debug
if (p[0].equals(n[0]) == false) {
postMsg(newMsg);
}
//println(s); // debug
}
댓글 없음:
댓글 쓰기